Information for Developers of Intelligent Tax Documents®
PDF versions of annual tax documents may "electronically deliver" tax data by embedding FDX JSON in the PDF "custom properties".
Such PDF files are referred to as intelligent tax documents®.
As seen below, the tax document information in FDX-standard JSON format is included internally in the PDF file.
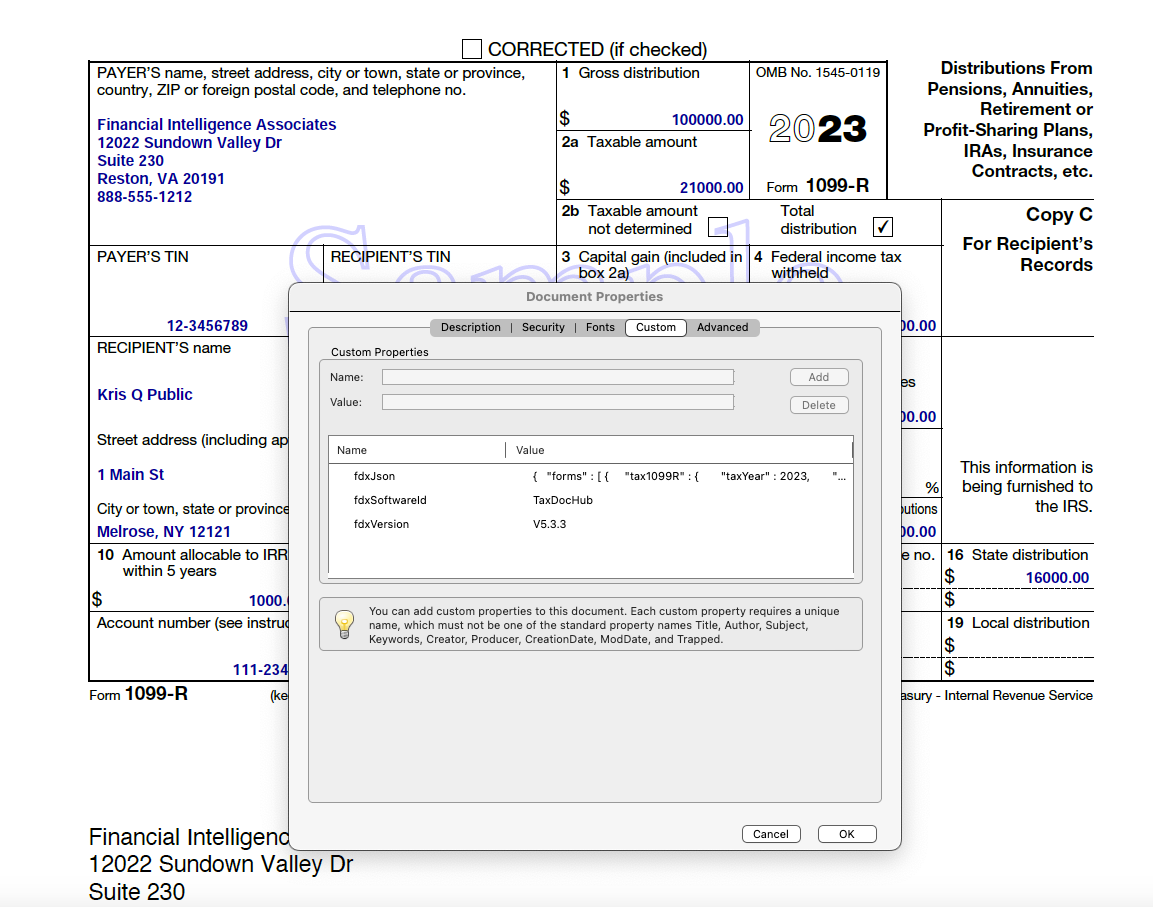
How to add FDX JSON to your PDF tax documents
Example Java code
import org.apache.pdfbox.pdmodel.PDDocument; import org.apache.pdfbox.pdmodel.PDDocumentInformation; import java.io.File; import java.io.IOException; public class PdfFdxJsonInjector { public void inject( String pdfInFilePath, String pdfOutFilePath, String fdxVersion, String fdxSoftwareId, String fdxJson ) throws IOException { try ( PDDocument document = PDDocument.load( new File( pdfInFilePath ) ); ) { // Inject custom properties PDDocumentInformation documentInformation = new PDDocumentInformation( ); documentInformation.setCustomMetadataValue( "fdxJson", fdxJson ); documentInformation.setCustomMetadataValue( "fdxVersion", fdxVersion ); documentInformation.setCustomMetadataValue( "fdxSoftwareId", fdxSoftwareId ); document.setDocumentInformation( documentInformation ); document.save( new File( pdfOutFilePath ) ); } catch ( Exception e ) { System.out.println( e.getMessage( ) ); } } }
How to read FDX JSON from PDF tax documents
Example Java code
import org.apache.pdfbox.pdmodel.PDDocument; import org.apache.pdfbox.pdmodel.PDDocumentInformation; import java.io.File; public class PdfFdxJsonReader { private String fdxJson; private String fdxVersion; private String fdxSoftwareId; public String getFdxJson( ) { return fdxJson; } public String getFdxVersion( ) { return fdxVersion; } public String getFdxSoftwareId( ) { return fdxSoftwareId; } public void read( String pdfInFilePath ) { try ( PDDocument document = PDDocument.load( new File( pdfInFilePath ) ); ) { PDDocumentInformation info = document.getDocumentInformation( ); if ( info == null ) { return; } this.fdxJson = info.getCustomMetadataValue( "fdxJson" ); this.fdxVersion = info.getCustomMetadataValue( "fdxVersion" ); this.fdxSoftwareId = info.getCustomMetadataValue( "fdxSoftwareId" ); } catch ( Exception e ) { System.out.println( e.getMessage( ) ); } } }